I am doing interviews few times a year, and it's probably not enough[1]. Opportunities aside, I learn a valuable lesson every time.
Interview pressure uncovers weaknesses. Mine are overcomplication, impatience and panic:
- I somehow expect the problem to be hard. So, I dismiss valuable cues and intuitions if they look too obvious.
- If I feel a hunch, I dive in without full understanding. I keep stepping on this rake... and solving a wrong problem!
- I the problem sounds intimidating, I panic and freeze, not knowing where to start.
Brute-force
To compensate for these weaknesses, I now start with a simplest solution that comes to mind. I work on a naïve solution first, clarifying my understanding, and then brainstorm ideas to improve it.
Structuring your work this way reduces the cognitive load when you start working on an improved solution.
This is an intuitive and well-recommended technique. However, I feel that candidates are afraid to look inexperienced and skip this step. As an interviewer, you may want to offer your candidate to start easy.
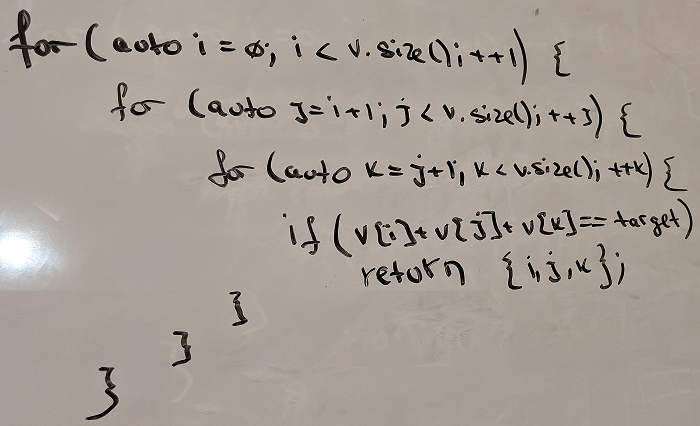
The Game Plan
It helps to mentally structure the interview into phases. The opening is important as it sets the tone, and brute-force can help you get through. Early phases are head-up: collaborate, learn and explore. Late phases are head-down: focus and deliver.
The game plan also helps manage your time and deliver a solution before the interview is over.